Gradient Descent
1차 미분 계수(기울기)를 이용해 함수의 최솟값을 찾는 방법
Gradient Descent는 함수의 최솟값을 찾는 방법 중 하나입니다. 함수의 기울기를 이용하여 최솟값을 찾습니다. Learning Rate는 학습 속도를 결정하는 요소 중 하나이며, 적절한 값을 선택하는 것이 중요합니다.
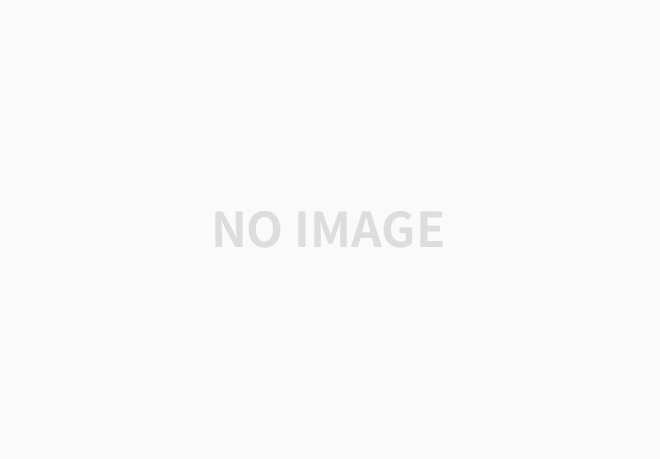
단순한 이차 함수의 최솟값을 Gradient Descent 알고리즘을 사용하여 찾아보겠습니다.
import numpy as np
# 이차 함수 정의: f(x) = x^2
def quadratic_function(x):
return x ** 2
# Gradient Descent 알고리즘 적용
def gradient_descent(starting_point, learning_rate, iterations):
x = starting_point
for i in range(iterations):
gradient = 2 * x # 이차 함수의 미분값은 2x
x = x - learning_rate * gradient
return x
# 시작점과 학습률 설정
starting_point = 5
learning_rate = 0.1
iterations = 100
# Gradient Descent로 최솟값 찾기
minimum_point = gradient_descent(starting_point, learning_rate, iterations)
print("Minimum point found by Gradient Descent:", minimum_point)
>>> Minimum point found by Gradient Descent: 1.0185179881672439e-09
Weight와 Bias
Weight는 신호 전달 세기(입력 데이터의 중요도)를 결정하고, Bias는 이전에 없던 추가적인 특성을 부여해줍니다. 둘 다 출력값에 영향을 미치는 요소입니다.
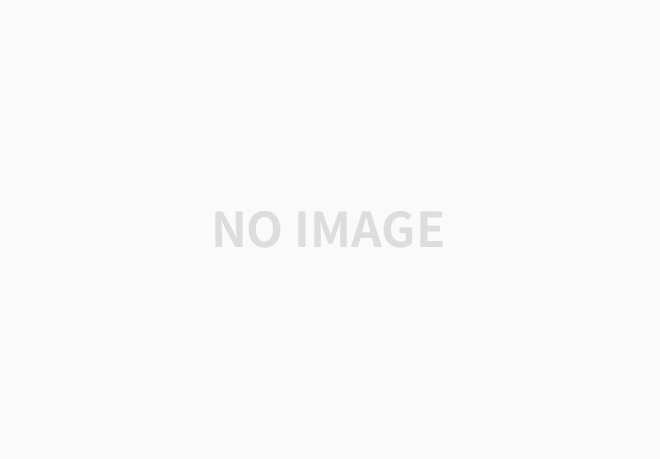
선형 회귀 모델에서 Weight와 Bias를 사용하여 데이터를 학습한다고 가정해보겠습니다.
import numpy as np
# 선형 회귀 모델의 예측 함수: y = wx + b
def linear_regression(x, weight, bias):
return weight * x + bias
# 입력 데이터
x = np.array([1, 2, 3, 4, 5])
# Weight와 Bias 설정
weight = 2
bias = 1
# 모델을 사용하여 예측값 계산
predictions = linear_regression(x, weight, bias)
print("Predictions:", predictions)
>>> Predictions: [ 3 5 7 9 11]
Overfitting과 Underfitting
Overfitting은 알고리즘이 학습한 데이터에 정확히 일치하도록 학습됐지만 원래 목적에는 맞지 않는 상태입니다. validation 데이터를 구축해서 학습하면서 loss 그래프의 추이를 통해 overfitting을 확인하고, 결과에 따라 학습 종료 조건을 이용해서 과대적합은 간접적으로 방지할 수 있습니다. 또는 모델의 layer를 수나 hidden layer의 크기를 줄여 모델이 과하게 복잡해지지 않도록 설계할 수 있습니다. Dropout을 통해 학습시 일부 뉴런을 누락하거나, data augmentation을 통해 학습 데이터를 늘릴 수 있습니다.
underfitting은 학습이 충분히 이루어지지 않은 상태를 의미합니다. epoch를 늘리거나 데이터의 특성에 맞게 model을 더 복잡하게 설계하거나, 데이터 양을 늘림으로 해결할 수 있습니다.
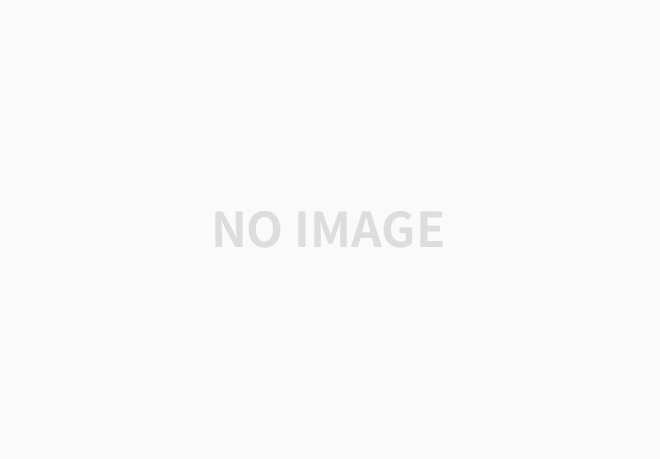
다음과 같은 다항식 회귀 모델을 사용하여 데이터를 학습한다고 가정해보겠습니다. 데이터가 실제로는 선형 관계를 가지고 있지만, 모델의 차수를 높여서 Overfitting과 Underfitting을 비교해보겠습니다.
import numpy as np
import matplotlib.pyplot as plt
from sklearn.preprocessing import PolynomialFeatures
from sklearn.linear_model import LinearRegression
from sklearn.pipeline import make_pipeline
# 데이터 생성
np.random.seed(0)
x = 2 - 3 * np.random.normal(0, 1, 20)
y = x - 2 * (x ** 2) + 0.5 * (x ** 3) + np.random.normal(-3, 3, 20)
# 선형 회귀 모델 적용
model = make_pipeline(PolynomialFeatures(1), LinearRegression())
model.fit(x[:, np.newaxis], y)
x_test = np.linspace(-5, 5, 100)
y_pred = model.predict(x_test[:, np.newaxis])
# 그래프 그리기
plt.figure(figsize=(10, 5))
plt.scatter(x, y, s=20, label='Training data')
plt.plot(x_test, y_pred, color='red', label='Linear fit (Underfitting)')
plt.xlabel('x')
plt.ylabel('y')
plt.legend()
plt.show()

'IT > AI' 카테고리의 다른 글
딥러닝 기초 핵심 개념 2 1 | 2024.03.11 |
---|---|
딥러닝 기초 핵심 개념 1 0 | 2024.03.08 |
인공 신경망 기초 0 | 2024.03.06 |
[이미지] CustomDataset [Python] - cv2, PIL 0 | 2023.09.07 |